It was my first blog in this platform where I mentioned how to retrieve data points from epm cloud applications. You can have a look at it from here. I have been thinking about writing how to update data points on epm cloud applications since my first blog and finally time has come to write more about it. Please note, I will give this as an example to update single data point but overall idea is that you can extend this example as a data push mechanism accross environments. For instance you have got one workforce application and another finance application in a different environment, you can push employee total cost to finance application on save for the edited cost centers and entities.
Lets start with this blank intersection.

If you want to update this grid programmatically, REST API is one of your routes to follow.You can send a request on the URL
https://domaindetails/HyperionPlanning/rest/v3/applications/applicationname/plantypes/cubename/importdataslice
Request body with JSON format should provide details of the grid that you are building up virtually. All the dimensions should be selected in either POV, Rows or Columns. See following JSON example to update the intersection which is same as smartview report above.
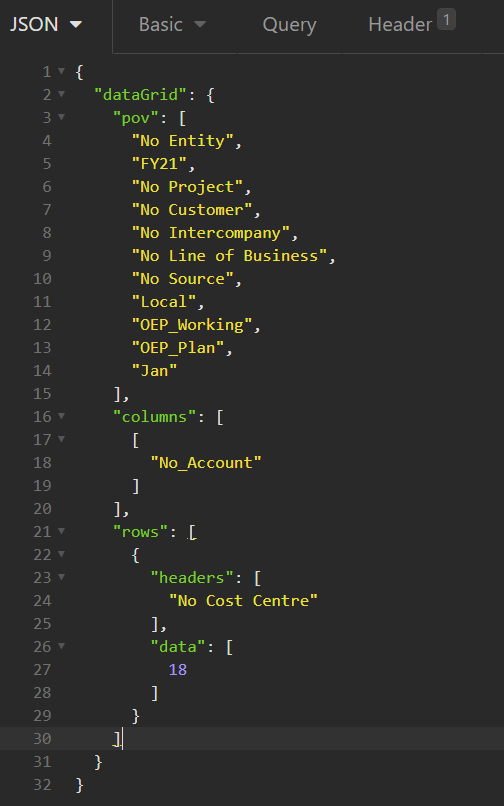
If everything is good, you will get the response as follows.
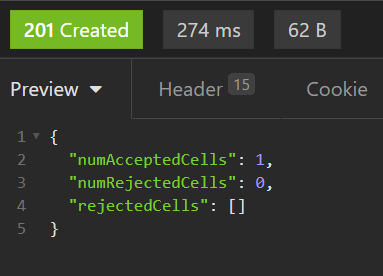
You can prepare same RestAPI query from a groovy script and let groovy script handle the execution. You will need to create the connection object, I won’t go into details of how to create importslice connection, please refer to my earlier blogs on more details on that.
def rows = new JSONObject() .put("headers",["No Cost Centre"]) .put("data",[18]) def cols = ["No_Account"] def importgrid = new JSONObject() .put("pov",["No Entity","FY21","No Project","No Customer","No Intercompany","No Line of Business","No Source","Local","OEP_Working","OEP_Plan","Jan"]) .put("columns", [cols]) .put("rows",[rows]) def body = new JSONObject() .put("dataGrid",importgrid) .toString() HttpResponse jsonResponse = operation.application.getConnection("importslice").post("/importdataslice").body(body).asString(); println(jsonResponse.body)
Once you launch the rule for this groovy rule with success, you will simply see same RestAPI response from job console.
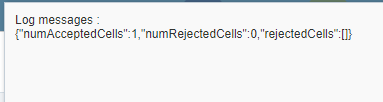
it is a good idea to check jsonResponse.status in an actual implementation and make sure it returns an OK status before you proceed processing what body response is returning. Real life examples may not always return status code like 200 which is http OK message. For instance, if there was an infrastructure issue or user had an expired password or similar situations would return an http error status that you will have to handle. Here is an example to check the status and throw an error in cases where http request is not successful.
if(!(200..299).contains(jsonResponse.status)){ throwvetoException("Error processing RestAPI: $jsonResponse.statusText") }
Going back to our grid in SmartView, it is updated as expected.
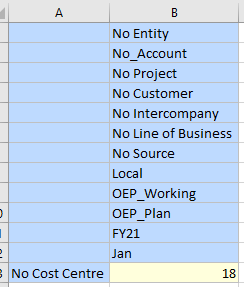
You can send multiple rows within the same RestAPI query to update multiple cells. You can read returning RestAPI response to see if any update fails.